1.引入依赖
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.9.8</version>
</dependency>
2.自定义一个注解
java">package com.example.springbootdemo3.annotation;
import java.lang.annotation.*;
@Target({ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@Documented
public @interface MyLog {
String name() default "";
}
3.自定义AOP
java">package com.example.springbootdemo3.aop;
import com.example.springbootdemo3.annotation.MyLog;
import org.aspectj.lang.ProceedingJoinPoint;
import org.aspectj.lang.annotation.Around;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Pointcut;
import org.aspectj.lang.reflect.MethodSignature;
import org.springframework.stereotype.Component;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.servlet.http.HttpServletRequest;
import java.lang.reflect.Method;
@Component
@Aspect
public class MyControllerAop {
@Pointcut("@annotation(com.example.springbootdemo3.annotation.MyLog)")
private void pointCut() {
}
@Around("pointCut()")
public Object around(ProceedingJoinPoint joinPoint) throws Throwable {
MethodSignature methodSignature = (MethodSignature) joinPoint.getSignature();
Method method = methodSignature.getMethod();
if (method != null) {
MyLog myLog = method.getAnnotation(MyLog.class);
System.out.println("MyLog name:" + myLog.name());
}
String name = method.getName();
System.out.println("方法名:" + name);
ServletRequestAttributes servletRequestAttributes = (ServletRequestAttributes) RequestContextHolder.getRequestAttributes();
HttpServletRequest request = servletRequestAttributes.getRequest();
String url = request.getRequestURL().toString();
System.out.println("访问url:" + url);
String method1 = request.getMethod();
System.out.println("请求方式:" + method1);
String queryString = request.getQueryString();
System.out.println("请求参数:" + queryString);
System.out.println("请求IP:" + getClientIpAddress(request));
Object proceed = joinPoint.proceed();
System.out.println("返回值:" + proceed);
return proceed;
}
private String getClientIpAddress(HttpServletRequest request) {
String ip = request.getHeader("X-Forwarded-For");
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("WL-Proxy-Client-IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_CLIENT_IP");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getHeader("HTTP_X_FORWARDED_FOR");
}
if (ip == null || ip.length() == 0 || "unknown".equalsIgnoreCase(ip)) {
ip = request.getRemoteAddr();
}
return ip;
}
}
3.写个测试Controller试一下
java">package com.example.springbootdemo3.config;
import com.example.springbootdemo3.annotation.MyLog;
import org.springframework.web.bind.annotation.PostMapping;
import org.springframework.web.bind.annotation.RequestBody;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.util.Map;
@RestController
@RequestMapping("/aop-test")
public class AopTestController {
@PostMapping("/test")
@MyLog(name = "aop测试")
public String aopTest(@RequestBody Map map) {
System.out.println(map);
return "success";
}
}
4.结束
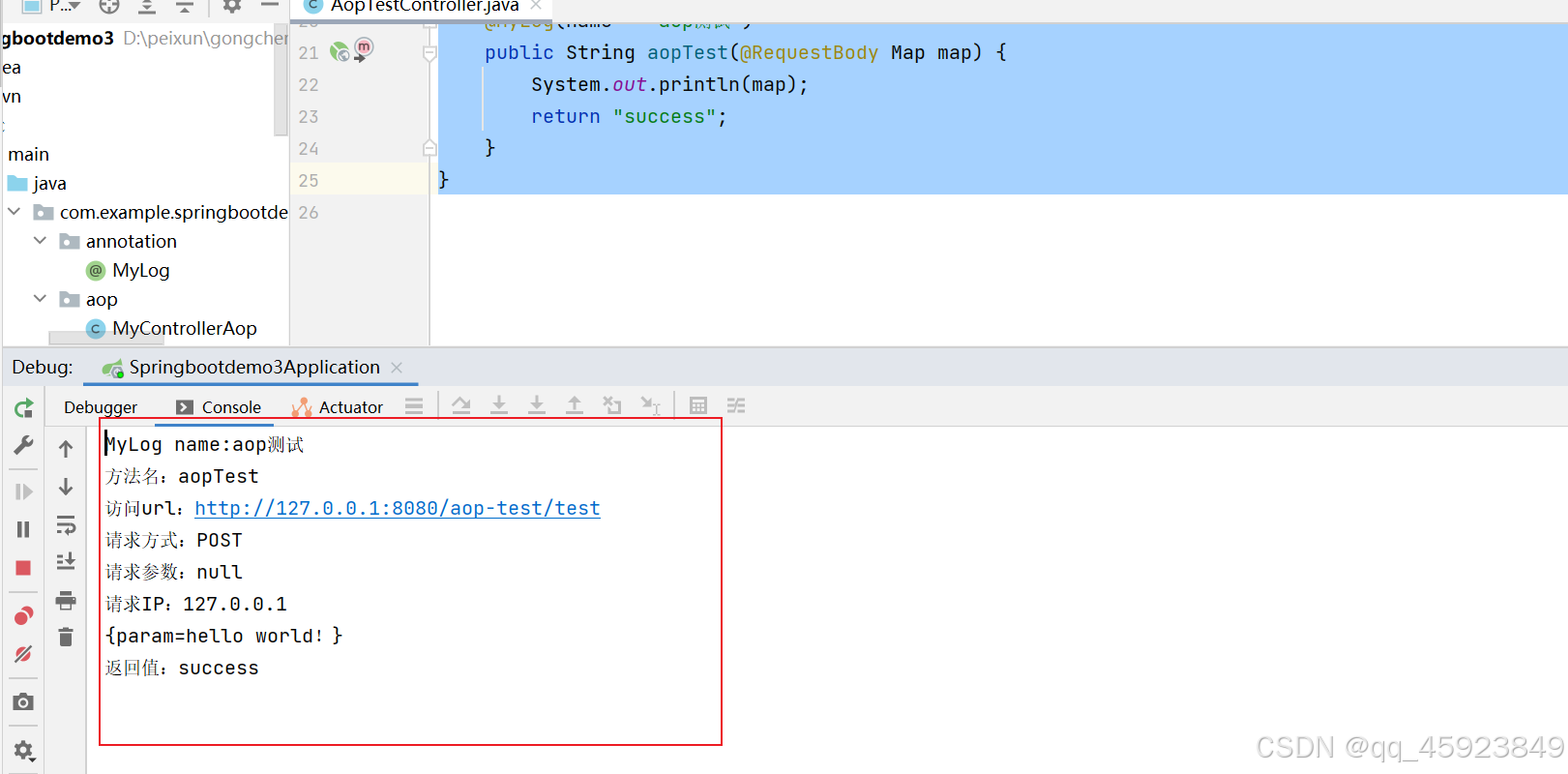